How to compile SDL3 application for emscripten (web) on linux with cmake
We are still gonna use the same test project as last time.
See the source code here: github
Installing toolchain
To compile for the web, we need emscripten installed:
$ cd ~ # We are going to install the toolchain in ~/emsdk/
$ git clone https://github.com/emscripten-core/emsdk.git && cd emsdk
$ ./emsdk install latest && ./emsdk activate latest
Compiling dependencies
We need SDL3 and SDL3_image, but emscripten doesn't provide these packages yet, thus we need to compile them by ourselfs
First, let's compile SDL
$ git clone https://github.com/libsdl-org/SDL && cd SDL
$ mkdir build && cd build
$ source ~/emsdk/emsdk_env.sh # Activate emscripten toolchain
$ emcmake cmake .. && emmake make && emmake install
Now let's compile SDL_image
$ git clone https://github.com/libsdl-org/SDL_image && cd SDL_image
$ mkdir build && cd build
$ source ~/emsdk/emsdk_env.sh # Activate emscripten toolchain
$ emcmake cmake -DBUILD_SHARED_LIBS=OFF .. && emmake make && emmake install # We need to disable shared libs for SDL_image to build
Compiling project
Now on to compiling the project!
$ git clone https://github.com/cheyao/TileMap
$ cd TileMap
$ mkdir build && cd build
$ source ~/emsdk/emsdk_env.sh # Activate emscripten toolchain
$ emcmake cmake .. && emmake make
Now we have TileMap.js!
In the project I included a demo index.html that get processed with cmake to fill in the project name
Here is the emscripten part of the CMakeLists.txt
find_package(SDL3 REQUIRED CONFIG REQUIRED COMPONENTS SDL3-static)
find_package(SDL3_image REQUIRED CONFIG REQUIRED COMPONENTS SDL3_image-static)
add_compile_options(-sUSE_SDL=3 -sUSE_SDL_IMAGE=3)
add_compile_options(${ASSETS_EMBED_WEB})
add_link_options(-sUSE_SDL=3 -sUSE_SDL_IMAGE=3)
add_link_options(${ASSETS_EMBED_WEB})
set(CMAKE_EXECUTABLE_SUFFIX .js)
configure_file(cmake/index.html.in ${CMAKE_BINARY_DIR}/index.html)
Now test it with python -m http.server 9000
to test it! Go to localhost:9000, or here for online demo
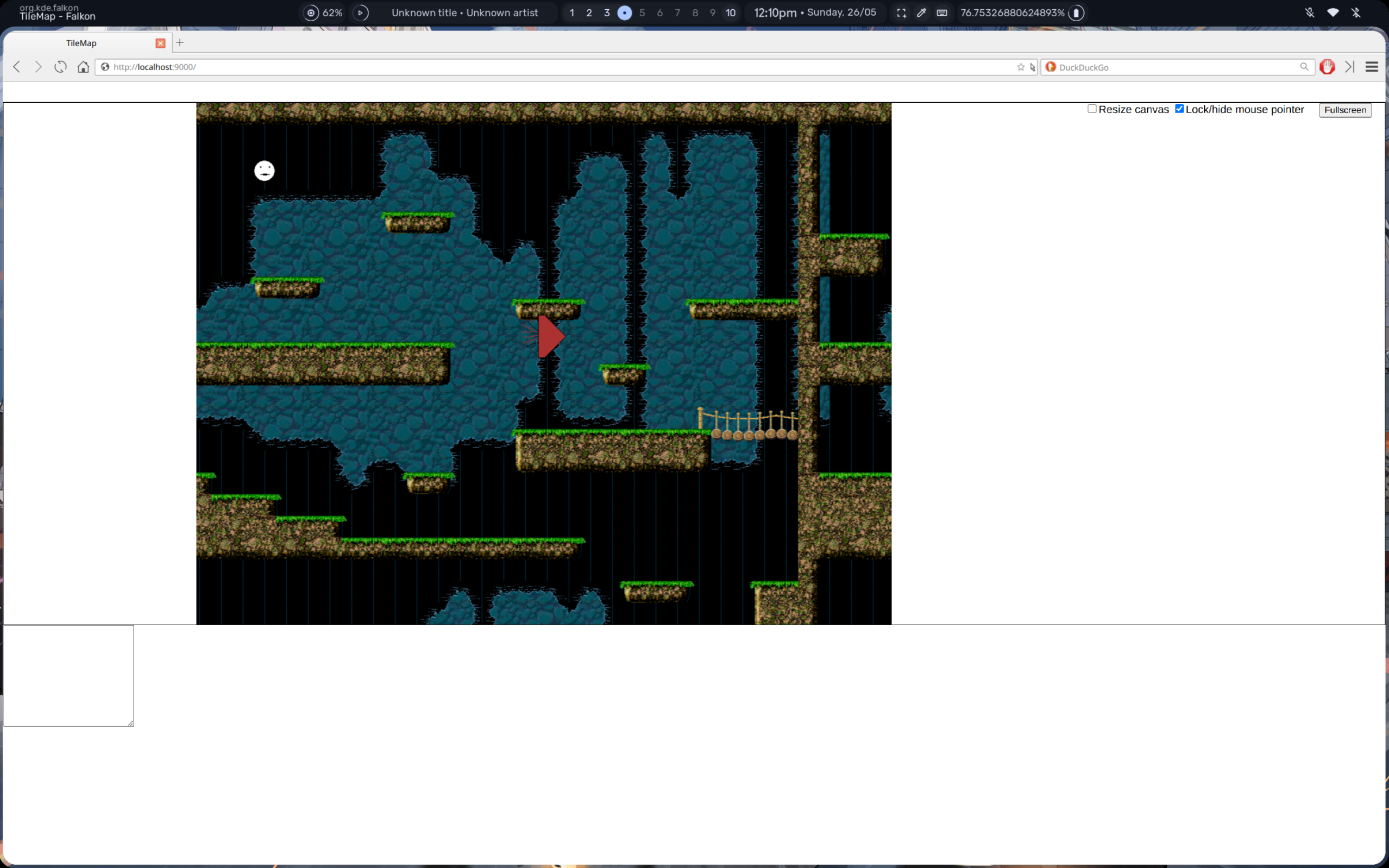